Hi Komoto,
perhaps I put too much emphasis on the Gif files.
In answer to your point, itās probably as short, as it is long e.g.
import wx
from ActiveBitmap import ActiveBitmap
class TestPanel(wx.Panel):
def __init__(self, *args, **kwargs):
super().__init__(*args, **kwargs)
ctrl = ActiveBitmap(self, -1, bitmap=wx.NullBitmap, bitmap_list=['led1s.gif',])
ctrl.SetTimerPeriod(50)
ctrl.Activate()
app = wx.App()
frm = wx.Frame(None)
frm.panel = TestPanel(frm)
frm.Show()
app.MainLoop()
But there are other advantages, because you can, in effect, create your own animations without a Gif file, simply by providing a list of images.
You can create a flashing animation by providing 2 images that toggle between each other.
Other adavantages are that unlike a standard Gif using AnimationCtrl, you can control the speed, the number of repetitions, or the period time to animate the images.
You can also divide the animation into ranges of images.
One option, not specifically mentioned but available is related to the fact that the supplied bitmaps are all resized to be the same size. This means that you can use ActiveBitmap as a dynamically resizing StaticBitmap i.e. throw any bitmap at it and specify a size=(x, y)
parameter and it will resize the image for you.
To achieve this you had to add a superfluous wx.NullBitmap
in the bitmap_list but I have made this easier to achieve in version 1.1.0. In this latest version you specify the bitmap with a size parameter and can omit the bitmap_list entirely.
Changelog:
1.1.0 Ensure that illegal values cannot be set in the SetRange function
Test for missing bitmap_list, allowing easier definition of a dynamic resizing bitmap,
rather than an animation
Don't start the period timer is there is only a single bitmap.
ActiveBitmap.py (26.6 KB)
Using ActiveBitmap as a resizing StaticBitmap
import wx
from ActiveBitmap import ActiveBitmap
class Frame(wx.Frame):
def __init__(self, parent):
wx.Frame.__init__(self, parent, -1, "ActiveBitmap Demonstration", size=(320, 450))
panel = wx.Panel(self)
box = wx.StaticBox(panel, wx.ID_ANY, "Using Active Bitmap for Dynamic resize", pos=(10, 10), size=(300, 400))
bitmap1 = ActiveBitmap(box, -1, pos=(10, 10), bitmap=wx.Bitmap('4.png'), size=(16, 16))
bitmap2 = ActiveBitmap(box, -1, pos=(10, 30), bitmap=wx.Bitmap('4.png'), size=(40, 40))
bitmap3 = ActiveBitmap(box, -1, pos=(10, 60), bitmap=wx.Bitmap('4.png'), size=(80, 80))
bitmap4 = ActiveBitmap(box, -1, pos=(10, 130), bitmap=wx.Bitmap('4.png'), size=(160, 80))
bitmap5 = ActiveBitmap(box, -1, pos=(40, 200), bitmap=wx.Bitmap('4.png'), size=(160, 160))
wx.StaticText(box, -1, "Image is actually 64 x 64", pos=(100, 10))
self.Show()
app = wx.App()
frame = Frame(None)
app.MainLoop()
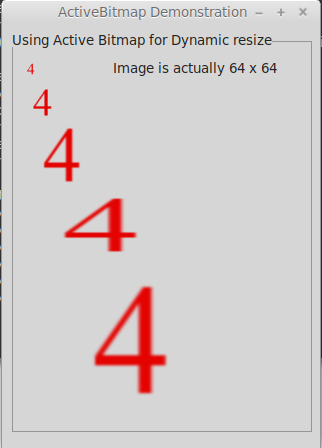
Finally, ActiveBitmap is not designed to replace AnimationCtrl, it can be an alternative with extra control or it can create quasi-animations from your own images.
As I said, it was based originally on a requirement to be able to flash a bitmap to denote that something relevant or of note had occurred, or as an alternative for a pulse progress dialog to denote something is happening.
I have a tendency to create software with message areas and/or indicator panels, this code allows me to pulse/change indicators, to keep the user informed about the state of processes/actions with a simple visual tool.
I hope that clears up what my intentions were, for this widget.
I apologise for any confusion.
Regards,
Rolf
p.s. Regards your throbber example: Ah! my eyes, my eyes, ⦠Zzzzzzzzzzzzzzz 