So basically, what is mentioned in the topic title. We need a widget that provides a multi-line plain-text editing control with a resize grabber.
This animation shows how this grabber should be used:
Does wxPython provide such a widget?
If not what would be the best starting point to develop such a widget?
Best thanks and greetings
Jaschar
Hi,
The simplest way to do this in wxPython is to use a wx.Frame containing a wx.TextCtrl (configured in multi-line mode) and using a wx.BoxSizer to manage the layout.
You can resize the Frame by dragging the edges or corners. On its own there is no visual indicator on the bottom right corner, but you can get that by adding a status bar to the Frame. The appearance may vary depending on operating system and/or theme.
Here is a simple example I created using the UI-builder wxGlade:
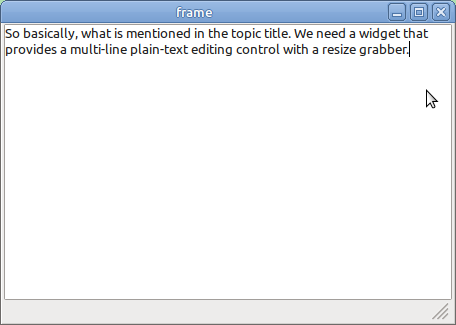
For more sophisticated editing needs there are also the wx.RichTextCtrl and wx.StyledTextCtrl.
An excellent way to see the controls available is to download the wxPython Demo application (see Index of /wxPython4/extras) that matches the version of wxPython that you have installed.
Code for the example:
#!/usr/bin/env python3
# -*- coding: UTF-8 -*-
#
# generated by wxGlade 1.0.5 on Tue Aug 22 17:44:42 2023
#
import wx
# begin wxGlade: dependencies
# end wxGlade
# begin wxGlade: extracode
# end wxGlade
class MyFrame(wx.Frame):
def __init__(self, *args, **kwds):
# begin wxGlade: MyFrame.__init__
kwds["style"] = kwds.get("style", 0) | wx.DEFAULT_FRAME_STYLE
wx.Frame.__init__(self, *args, **kwds)
self.SetSize((456, 325))
self.SetTitle("frame")
self.frame_statusbar = self.CreateStatusBar(1)
self.frame_statusbar.SetStatusWidths([-1])
self.panel_1 = wx.Panel(self, wx.ID_ANY)
sizer_1 = wx.BoxSizer(wx.VERTICAL)
self.text_ctrl_1 = wx.TextCtrl(self.panel_1, wx.ID_ANY, "So basically, what is mentioned in the topic title. We need a widget that provides a multi-line plain-text editing control with a resize grabber.", style=wx.TE_MULTILINE)
sizer_1.Add(self.text_ctrl_1, 1, wx.EXPAND, 0)
self.panel_1.SetSizer(sizer_1)
self.Layout()
# end wxGlade
# end of class MyFrame
class MyApp(wx.App):
def OnInit(self):
self.frame = MyFrame(None, wx.ID_ANY, "")
self.SetTopWindow(self.frame)
self.frame.Show()
return True
# end of class MyApp
if __name__ == "__main__":
app = MyApp(0)
app.MainLoop()
Tested using Python 3.10.12 + wxPython 4.2.1 gtk3 (phoenix) wxWidgets 3.2.2.1 on Linux Mint 21.2
Are you aware of wx.lib.expando.ExpandoTextCtrl
? It’s not the specific thing you asked for, but depending on your precise use case, it might be an acceptable alternative.