In your example image the outer ring of the speedmeter gets lighter at the left and right edges. I had a play with the GraphicsContext
class to see if I could create something similar.
The code below is a quick hack that just draws the outer ring that fades in a similar manner. It might provide some ideas.
I have only tested it using Python 3.10.12 + wxPython 4.2.1 gtk3 (phoenix) wxWidgets 3.2.2.1 on Linux Mint 21.2
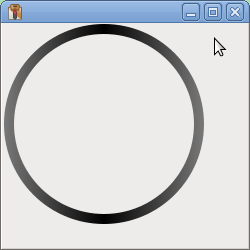
import math
import wx
class GradientRing(wx.Control):
def __init__(self, parent, inner_radius, outer_radius, inner_colour, outer_colour):
super(GradientRing, self).__init__(parent, style=wx.NO_BORDER)
self.inner_radius = inner_radius
self.outer_radius = outer_radius
self.inner_colour = inner_colour
self.outer_colour = outer_colour
self.Bind(wx.EVT_PAINT, self.OnPaint)
def DoGetBestSize(self):
return wx.Size(self.outer_radius*2, self.outer_radius*2)
def OnPaint(self, _event):
gcdc = wx.GCDC(wx.PaintDC(self))
gc = gcdc.GetGraphicsContext()
pen = gc.CreatePen(wx.TRANSPARENT_PEN)
gc.SetPen(pen)
x1 = self.outer_radius
y1 = self.outer_radius
# Draw right segment
grad_brush = gc.CreateLinearGradientBrush(x1, y1,
x1*2, y1,
self.inner_colour,
self.outer_colour)
gc.SetBrush(grad_brush)
path = gc.CreatePath()
path.AddArc(x1, y1, self.outer_radius, math.radians(270), math.radians(90), True)
path.AddArc(x1, y1, self.inner_radius, math.radians(270), math.radians(90), True)
gc.DrawPath(path)
# Draw left segment
grad_brush = gc.CreateLinearGradientBrush(x1, y1,
0, y1,
self.inner_colour,
self.outer_colour)
gc.SetBrush(grad_brush)
path = gc.CreatePath()
path.AddArc(x1, y1, self.outer_radius, math.radians(270), math.radians(90), False)
path.AddArc(x1, y1, self.inner_radius, math.radians(270), math.radians(90), False)
gc.DrawPath(path)
class MyPanel(wx.Panel):
def __init__(self, parent):
super(MyPanel, self).__init__(parent)
vsizer = wx.BoxSizer(wx.VERTICAL)
colour_1 = wx.Colour(0, 0, 0)
colour_2 = wx.Colour(120, 120, 120)
ring = GradientRing(self, 90, 100, colour_1, colour_2)
vsizer.Add(ring, 0, 0, 0)
self.SetSizer(vsizer)
class MyFrame(wx.Frame):
def __init__(self, parent):
super(MyFrame, self).__init__(parent)
self.SetSize((250, 250))
self.panel = MyPanel(self)
if __name__ == '__main__':
app = wx.App()
frame = MyFrame(None)
frame.Show()
app.MainLoop()