So the problem is the keybindings changed from windows 7 to windows 10.
Here is where i checked: https://support.microsoft.com/en-us/help/12445/windows-keyboard-shortcuts
If you look on Windows 10 the key you are trying to bind to is reserved in Windows 10 as show in the picture:
What i suggest is verify that that the key you are trying to bind to is not already taken by checking the return value from the self.RegisterHotKey(...)
function. It will return true if it was successfull and false if something already has it. See documentation here: https://wxpython.org/Phoenix/docs/html/wx.Window.html?highlight=registerhotkey#wx.Window.RegisterHotKey.
Since wxpython is binding globally it is whatever keys are registered to the OS in this case Windows 10 vs Windows 7. The OS handles these events and get registered with it so it knows what to look for and who to call when the keys are seen.
Here is sample code I drafted up for python3 to check if it is valid by adding a print statement:
import wx
import win32con #for the VK keycodes
# Got this class from example here: https://wiki.wxpython.org/RegisterHotKey
class FrameWithHotKey(wx.Frame):
def __init__(self, *args, **kwargs):
wx.Frame.__init__(self, *args, **kwargs)
self.regHotKeys()
self.Bind(wx.EVT_HOTKEY, self.handleHotKey, id=self.hotKeyId)
self.Bind(wx.EVT_HOTKEY, self.handleHotKey, id=self.hotKeyId+1)
def regHotKeys(self):
"""
This function registers the hotkey Alt+F1 with id=100
"""
self.hotKeyId = 100
# output will be False due to binding already in Windows 10!!!
# WIN + J
print(self.RegisterHotKey(self.hotKeyId, win32con.MOD_WIN,74) ) # 'J'
# output will be True now because none of my programs / Windows use CTRL + J
print(self.RegisterHotKey(self.hotKeyId+1, win32con.MOD_CONTROL,74) ) # 'J'
def handleHotKey(self, evt):
"""
Prints a simple message when a hotkey event is received.
"""
print("do hot key actions")
def main():
app = wx.App(False) # Create a new app, don't redirect stdout/stderr to a window.
frame = FrameWithHotKey(None, wx.ID_ANY, "Menu Testing") # A Frame is a top-level window.
frame.Show(True) # Show the frame.
app.MainLoop()
if __name__ == "__main__":
main()
Sample Output: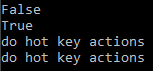
I wish there was an easy answer but it comes down to check what is taken already based on everything that is installed. Microsoft shows a way to change Windows Shortcuts here: https://support.microsoft.com/en-us/help/4052277/accessories-how-do-i-reassign-hot-keys-for-my-keyboard but I haven’t tested it just an FYI.
Got to love Microsoft… 
And to answer why AutoHotKey works is because it overrides these somehow based on my understanding. I don’t know all the details but this is based on some quick research and WIKI.
Let me know if this answers everything!